Using {eac}Doojigger's "Advanced Mode" to control options and features.
Document Header
Homepage:https://eacDoojigger.earthasylum.com/
Author:EarthAsylum Consulting
Last Updated:09-May-2025
Contributors:EarthAsylum Consulting, Kevin Burkholder
Requires {eac}Doojigger:3.1.2
WordPress URI:https://wordpress.org/plugins/search/earthasylum/
GitHub URI:https://github.com/EarthAsylum/docs.eacDoojigger/wiki/
Implementing and Using Advanced Mode
{eac}Doojigger's Advanced Mode is a small set of tools that can be used by Doojigger (derivative) plugins to control or limit acces to options and/or features. In it's simplest form, Advanced Mode is a toggle, either on or off, and options or features may be made available or not based on that toggle.
How the toggle is set (or even if it is supported) as well as the ability to use multiple toggles or levels is much up to the developer.
{eac}Doojigger provides an array for a "global" setting and a "settings" setting, the latter pertaining to administrator options seen on the plugin's options page.
public $advanced_mode = array(
'global' => array(
'default' => false,
),
'settings' => array(
'default' => false,
),
);
Derivative plugins may extend this array as needed to provide Advanced Mode settings for any number of features or simply use the array as-is.
Since version 3 of {eac}Doojigger, the user roles are included in the global
and settings
arrays. For example, when an administrator is logged in, $advanced_mode['global']['administrator']
will be true;
The settings
array is used when an administrator option field includes the advanced
meta attribute:
// only available when advanced mode is enabled
'myTextField' => array(
'type' => 'textarea',
'label' => 'A text field',
'advanced' => true,
);
Methods
There are 3 methods available to support Advanced Mode...
public function allowAdvancedMode(bool $allow = null): bool
public function setAdvancedMode( $is = true, string $what = null, string $level = null): void
public function isAdvancedMode(string $what = null, string $level = null): bool
To enable support for Advanced Mode, a plugin first calls allowAdvancedMode()
:
$this->allowAdvancedMode( true );
This enables support but does not enable Advanced Mode.
To turn the Advanced Mode toggle on, use setAdvancedMode()
:
$this->setAdvancedMode( true );
$this->setAdvancedMode( true, 'global' );
$this->setAdvancedMode( true, 'global', 'default' );
The above 3 statements all do the same thing, turn the default global toggle on. To enable Advanced Mode for settings:
$this->setAdvancedMode( true, 'settings' );
$this->setAdvancedMode( true, 'settings', 'default' );
To extend options, say, for settings
based on a license level:
$this->setAdvancedMode( true, 'settings', 'professional' );
Then we can use "professional" with the "advanced" field meta attribute
'advanced' => 'professional',
so that a field is only accessable when ['settings']['professional']
is true.
The third method, isAdvancedMode()
, is used to check for Advanced Mode:
if ( $this->isAdvancedMode() ) {...}
if ( $this->isAdvancedMode( 'global' ) ) {...}
if ( $this->isAdvancedMode( 'global', 'default' ) ) {...}
As with setAdvancedMode()
, these 3 statements do the same thing... check the ['global']['default']
value. To check a settings
value:
if ( $this->isAdvancedMode( 'settings' ) ) {...}
if ( $this->isAdvancedMode( 'settings', 'default' ) ) {...}
if ( $this->isAdvancedMode( 'settings', 'professional' ) ) {...}
Filters
The allow_advanced_mode
filter can be used to control Advanced Mode from a higher level. This filter may be used to enable or disable Advanced Mode as a whole based on the criteria necessary for your plugin. The allow_advanced_mode
filter is triggered by the allowAdvancedMode()
method.
$this->add_filter('allow_advanced_mode', array( $this, 'allow_advanced_mode'));
$this->allowAdvancedMode( true );
public function allow_advanced_mode(bool $allow): bool
{
return $allow && $this->isProfessionalLicense();
}
The is_advanced_mode
filter can be used like the isAdvancedMode()
method.
$advancedMode = $this->apply_filters('is_advanced_mode',false,'settings');
Constants
{eac}Doojigger, in its abstract class, checks for a {pluginName}_ADVANCED_MODE
constant set in wp-config.php. If set to true, ['global']['default']
and ['settings']['default']
are set to true.
Custom Settings
Any additional settings must be handled by the derivative plugin. The easiest way to do this is by overloading the setAdvancedMode()
method:
public function setAdvancedMode( $is = true, string $what = null,string $level = null): void
{
if ($is === true && $what == 'settings')
{
$this->advanced_mode['settings']['standard'] = $this->isStandardLicense();
$this->advanced_mode['settings']['professional'] = $this->isProfessionalLicense();
$this->advanced_mode['settings']['enterprise'] = $this->isEnterpriseLicense();
}
parent::setAdvancedMode($is,$what,$level);
}
When custom settings liike this are used, rather than removing the setting when setAdvancedMode()
returns false, the setting field is replaced with a display field indicating that the setting is not available.
- The default display is "{level} Level Feature" (e.g. "Professional Level Feature").
- If the level starts with "-" (e.g. "-professional") the field is removed, not displayed.
- If the level has more than one word (e.g. "Professional License Required") the value is displayed as-is.
- In these examples:
- "professional", "Professional", and "-professional" are the same.
- "Professional License Required" is unique.
Toggling Advanced Mode
If/when $this->allowAdvancedMode()
returns true, a clickable link is added to the plugin settings page so that Advanced Mode can be enabled (Advanced) or disabled (Essentials) from the page.
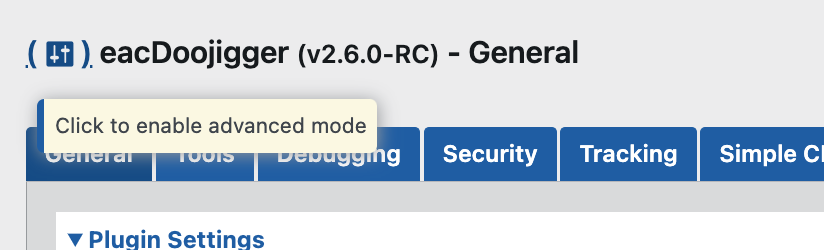
A similar link can be added elseware, such as a menu or button action, by using the add_admin_action_link()
method:
$href = $this->add_admin_action_link( 'enable_advanced_mode' );
$href = $this->add_admin_action_link( 'disable_advanced_mode' );
This add_admin_action_link()
url is handled by the {eac}Doojigger abstract class by triggering the action passed to it (enable_advanced_mode
/ disable_advanced_mode
).
Important Notes
Advanced Mode is a user preference enabled or disabled on a per-user basis. The {pluginName}_ADVANCED_MODE
constant set in wp-config.php overrides the user preference.
isAdvancedMode()
bubbles up when checking for a true|false value.
If calling $this->isAdvancedMode('MyFeature','MyLevel')
and ['MyFeature']['MyLevel']
is not set, ['MyFeature']['default']
is checked. If ['MyFeature']['default']
is not set, ['global']['MyLevel']
or ['global']['default']
is checked.
This means, for example, if checking a field attribute for a custom mode...
'myTextField' => array(
'type' => 'textarea',
'label' => 'A text field',
'advanced' => 'professional',
);
... and ['settings']['professional']
has not been set, the result will be that of ['settings']['default']
.
If setting a custom mode with $this->setAdvancedMode(bool,'MyFeature','MyLevel')
, and ['MyFeature']['default']
is not set, ['MyFeature']['default']
will be set to the opposite of ['MyFeature']['MyLevel']
.